Better URLs with Struts2
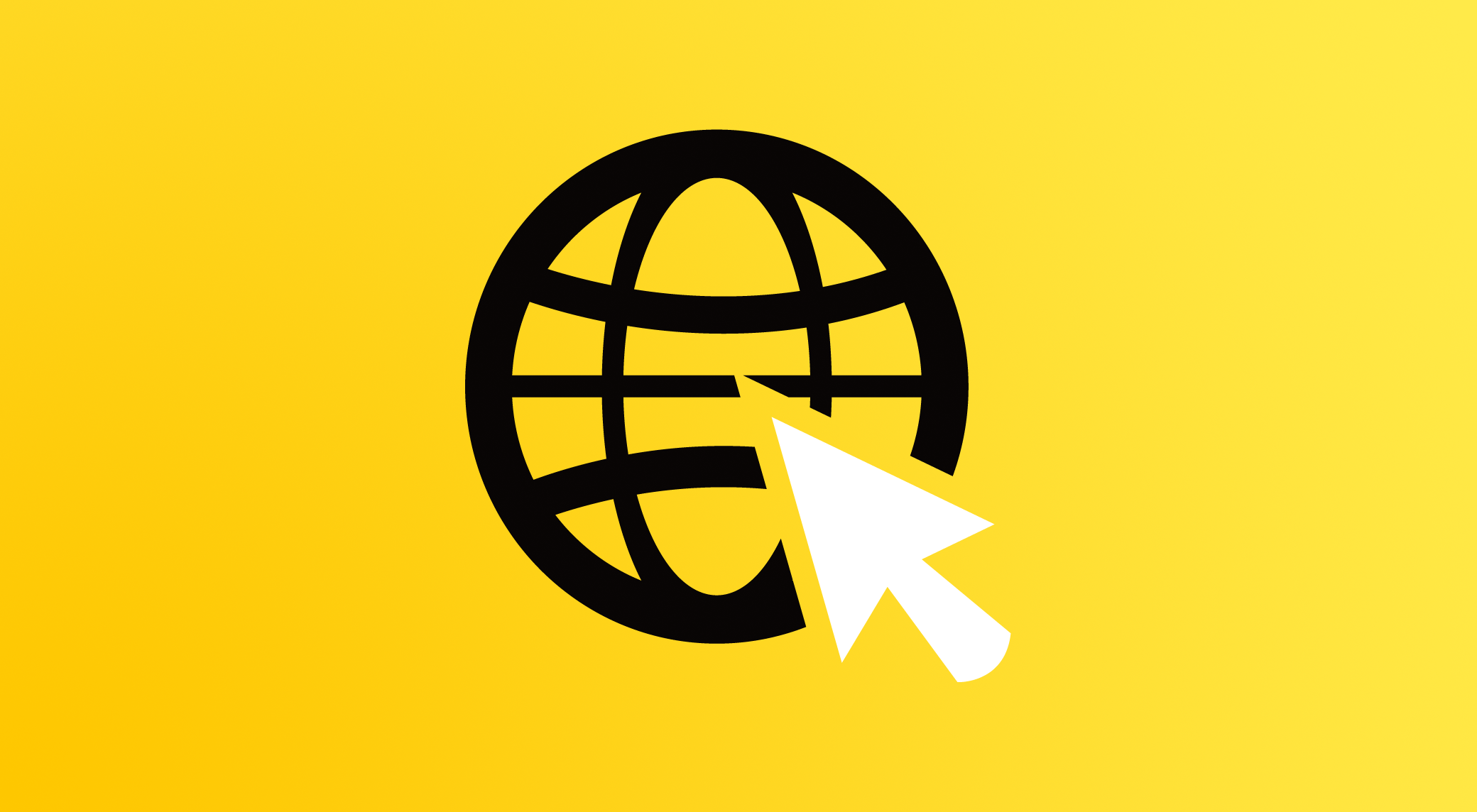
I’m going to show you how to use the NamedVariablePatternMatcher
to build better URLs with Struts2.
Before: http://www.example.com/profiles?username=steven
After: http://www.example.com/profiles/steven
For this example, we’ll map an action that displays a user’s profile page.
The Action
public class ViewProfileAction {
private String username;
public String execute() {
// look up the appropriate user by username and
// expose the user to the JSP with a getUser() method.
}
@RequestParameter
public void setUsername(String username) {
this.username = username;
}
}
The Standard Mapping
<action name="profiles" class="com.example.actions.ViewProfileAction">
<result name="input">/WEB-INF/jsp/profile.jsp</result>
</action>
The Improved Mapping
First, we need to set a few constants in our struts.xml file to enable named variable pattern matching.
<constant name="struts.mapper.alwaysSelectFullNamespace" value="false"/>
<constant name="struts.enable.SlashesInActionNames" value="true"/>
<constant name="struts.patternMatcher" value="namedVariable"/>
Next, we’ll revise the action mapping as follows.
<action name="profiles/{username}" class="com.example.actions.ViewProfileAction">
<result name="input">/WEB-INF/jsp/profile.jsp</result>
</action>
For the URL profiles/steven
, Struts2 will invoke our ViewProfileAction
and pass the value steven
to the setUsername
method of the action.
Happy action mapping!
Important Note
Only one PatternMatcher implementation can be used at a time. The two implementations included with Struts2 are mutually exclusive. You cannot use wildcards and named variable patterns in the same application (if that were required, you’d need to create a custom PatternMatcher implementation).